Streamlitは簡単にウェブアプリを作成できるPythonライブラリです。
アプリを見栄え良くするためにアイコンを使いたい場合、無料で豊富な種類を持つ Bootstrap Icons が便利です。
本記事では、StreamlitでBootstrap Iconsを使う方法についてまとめます。
Bootstrap Iconsとは?
Bootstrap Icons は、Bootstrapフレームワークで使用される公式のオープンソースアイコンライブラリです。
現在、1,600種類以上のアイコン が用意されており、SVGやWebフォントとしても利用可能です。
これらのアイコンは、データの可視化やボタン、メニューなど、さまざまなUI要素に使えます。
公式サイト
Bootstrap Icons · Official open source SVG icon library for Bootstrap
StreamlitでBootstrap Iconsを使う方法
Streamlitでは、st.markdown
と HTML を組み合わせることで、簡単にBootstrap Iconsを利用できます。
アイコンの使い方
StreamlitではデフォルトでBootstrap Iconsをサポートしていません。
そのため、CDN(Content Delivery Network) を使って読み込みます。
import streamlit as st
st.markdown("""
<link rel="stylesheet" href="https://cdn.jsdelivr.net/npm/bootstrap-icons@1.10.5/font/bootstrap-icons.css">
""", unsafe_allow_html=True)
読み込みが完了したら、<i>
タグでアイコンを表示できます。
st.markdown('<i class="bi bi-person"></i> ユーザー', unsafe_allow_html=True)
st.markdown('<i class="bi bi-chat-square-text"></i> メッセージ', unsafe_allow_html=True)
実行結果
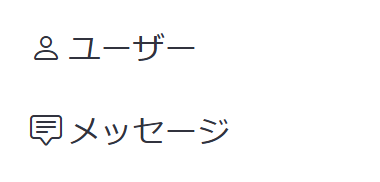
streamlit-option-menuでBootstrap Iconsを使う方法
streamlit-option-menu
は、ナビゲーションメニューを作るためのStreamlit拡張ライブラリです。
このライブラリでは、icons オプションでBootstrap Iconsを指定可能です。
使い方
事前にライブラリをインストールします。
pip install streamlit-option-menu
サンプルコードです。
from streamlit_option_menu import option_menu
import streamlit as st
selected = option_menu(
None,
["ホーム", "プロフィール", "メッセージ"],
icons=["house", "person", "chat-square-text"],
menu_icon="cast",
default_index=0
)
st.write(f"選択されたメニュー: {selected}")
実行結果
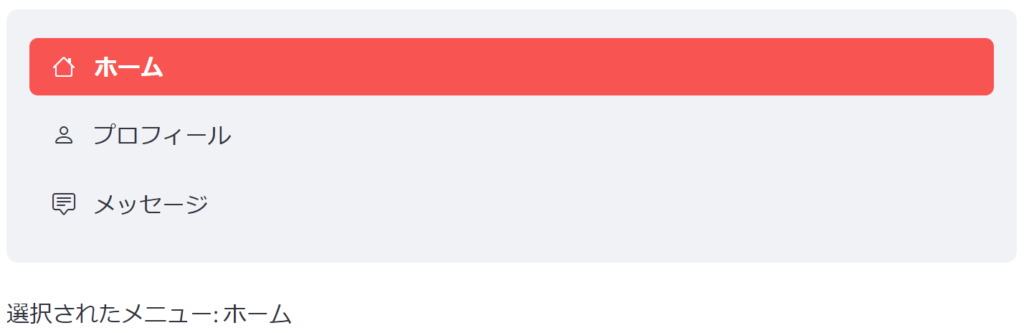
ポイント
icons
で指定するアイコン名は公式サイトのクラス名 (bi bi-アイコン名
) の bi-
を除いた部分です。
Bootstrap Iconsの一覧を確認するPythonコード
Streamlitで使えるBootstrap Iconsの一覧を確認する簡単なPythonコードです。
アイコンそのものと名称をStreamlitで表示するため、どのアイコンがどんな見た目かを一目で確認できます。
import requests
from bs4 import BeautifulSoup
import streamlit as st
# Bootstrap IconsのCSSを読み込み
st.markdown("""
<link rel="stylesheet" href="https://cdn.jsdelivr.net/npm/bootstrap-icons@1.10.5/font/bootstrap-icons.css">
""", unsafe_allow_html=True)
# Bootstrap Iconsのページからアイコン名を取得
url = "https://icons.getbootstrap.com/"
response = requests.get(url)
soup = BeautifulSoup(response.content, "html.parser")
# アイコンのクラス名と名称を抽出
icons = soup.select(".bi")
icon_names = [icon['class'][1] for icon in icons]
# アイコン名からジャンルを推測(カテゴリごとに分類)
categories = {
"矢印": [icon for icon in icon_names if "arrow" in icon],
"人": [icon for icon in icon_names if "person" in icon or "people" in icon],
"ファイル": [icon for icon in icon_names if "file" in icon],
"チャット": [icon for icon in icon_names if "chat" in icon or "message" in icon],
"その他": [icon for icon in icon_names if not any(keyword in icon for keyword in ["arrow", "person", "people", "file", "chat", "message"])]
}
# ジャンルの選択肢を作成
category = st.sidebar.selectbox("表示するジャンルを選んでください", list(categories.keys()))
# ページネーション設定
icons_per_page = 50
total_pages = -(-len(categories[category]) // icons_per_page) # 天井関数でページ数計算
# 現在のページをセッションステートで管理(初期値は1)
if 'page' not in st.session_state:
st.session_state.page = 1
# ページ切り替えボタン
col1, col2 = st.columns(2)
with col1:
if st.button("⬅️ 前のページ"):
if st.session_state.page > 1:
st.session_state.page -= 1
with col2:
if st.button("次のページ ➡️"):
if st.session_state.page < total_pages:
st.session_state.page += 1
# 現在のページに応じたアイコンを表示
start = (st.session_state.page - 1) * icons_per_page
end = start + icons_per_page
display_icons = categories[category][start:end]
st.write(f"### {category} のアイコン ({start + 1} 〜 {min(end, len(categories[category]))} / {len(categories[category])})")
# アイコンと名前を表示
for icon_name in display_icons:
st.markdown(f'<i class="bi {icon_name}" style="font-size:24px; margin-right:10px;"></i> `{icon_name}`', unsafe_allow_html=True)
# 現在のページ情報を表示
st.write(f"ページ {st.session_state.page} / {total_pages}")
実行結果
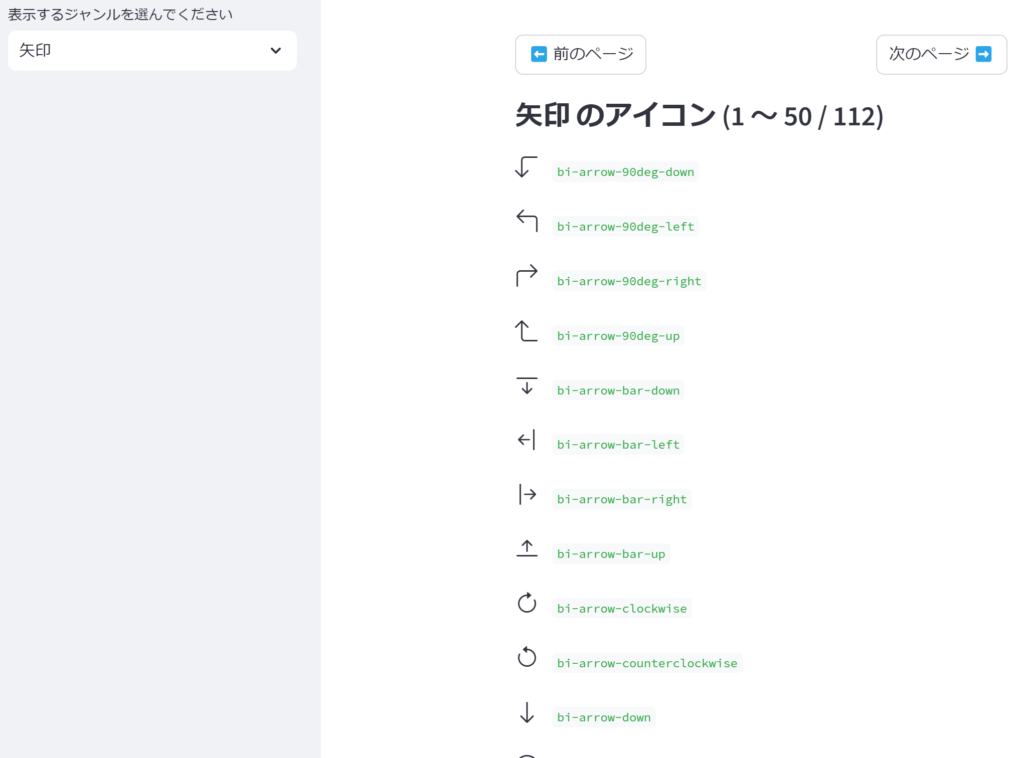
コードのポイント
- Bootstrap IconsのCSSを読み込み
st.markdown
で Bootstrap Icons の CDN を読み込み、Streamlit上でアイコンを使えるようにしています。- アイコンは
<i class="bi アイコン名"></i>
形式で表示。
- ジャンルごとにアイコンを分類
矢印
,人
,ファイル
,チャット
,その他
のカテゴリにアイコンを分類。- アイコン名に含まれるキーワード (
arrow
,person
,file
など) で自動的にカテゴリ分け。 - これにより、ユーザーが特定のジャンルのアイコンだけを効率的に閲覧可能。
- ページ切り替えをボタンで実装
st.button
を使い、前のページ と 次のページ ボタンを設置。- ボタンをクリックすると
st.session_state.page
が増減し、表示されるアイコンも切り替え。
- 1ページあたりのアイコン数を指定
icons_per_page
を 50 に設定し、50個ずつアイコンを表示。- ページごとにアイコンの表示位置を計算し、スムーズに切り替え。
- 現在のページをセッションで管理
st.session_state.page
で現在のページ情報を保持し、ボタンを押してもページがリセットされない。- アイコンの表示が安定するように工夫。
- アイコンと名前を見やすく表示
<i>
タグでアイコンを表示し、インラインCSSでサイズ (font-size:24px
) や余白 (margin-right
) を調整。- アイコンの横にクラス名 (
bi-アイコン名
) を表示し、ユーザーが簡単にコピペできるように工夫。
- サイドバーでジャンルを選択
st.sidebar.selectbox
でジャンルを選べるようにし、特定のアイコンだけをフィルタリング。- 選択したジャンルに応じて、該当するアイコンだけを効率的に表示。
Bootstrap Iconsのカスタマイズ
Streamlitでアイコンの色やサイズをカスタマイズするには、CSS を使います。
import streamlit as st
# Bootstrap IconsのCSSを読み込み
st.markdown("""
<link rel="stylesheet" href="https://cdn.jsdelivr.net/npm/bootstrap-icons@1.10.5/font/bootstrap-icons.css">
""", unsafe_allow_html=True)
# カスタムスタイルとデフォルトスタイルのCSS
st.markdown("""
<style>
.custom-icon {
color: #FF6347; /* オレンジ色に変更 */
font-size: 24px; /* サイズを24pxに変更 */
}
.default-icon {
color: #000; /* デフォルトの黒色 */
font-size: 20px; /* デフォルトサイズ */
}
</style>
""", unsafe_allow_html=True)
# アイコン比較の表示
st.write("### アイコンの変更前後の比較")
# 比較するアイコン一覧
icon_list = ["bi-gear", "bi-alarm", "bi-chat-square-text", "bi-star", "bi-person"]
# すべてのアイコンを変更前後で並べて表示
for icon in icon_list:
st.markdown(f"""
<div style="display: flex; align-items: center; margin-bottom: 10px;">
<div style="width: 50%; text-align: center;">
<i class="{icon} default-icon"></i> <code>{icon}(デフォルト)</code>
</div>
<div style="width: 50%; text-align: center;">
<i class="{icon} custom-icon"></i> <code>{icon}(カスタム)</code>
</div>
</div>
""", unsafe_allow_html=True)
実行結果
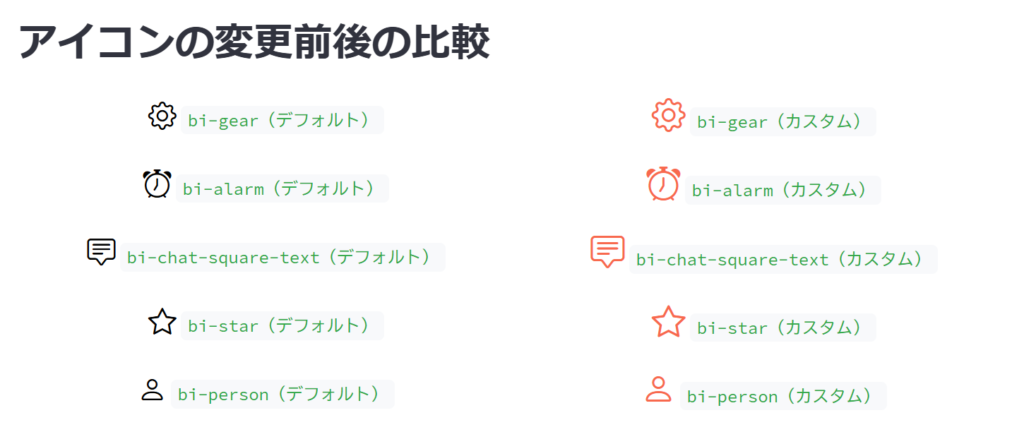
まとめ
- Bootstrap Icons は無料で使える豊富なアイコンセット。
st.markdown
で簡単にStreamlitに組み込み可能。streamlit-option-menu
なら、メニューアイコンも簡単に設定できる。- アイコンのカスタマイズもCSSで柔軟に対応。
これで、Streamlitアプリにアイコンを使って見た目をアップグレードできます。